Python进阶小技巧
懂得这些小技巧,也许能让代码看起来更简洁
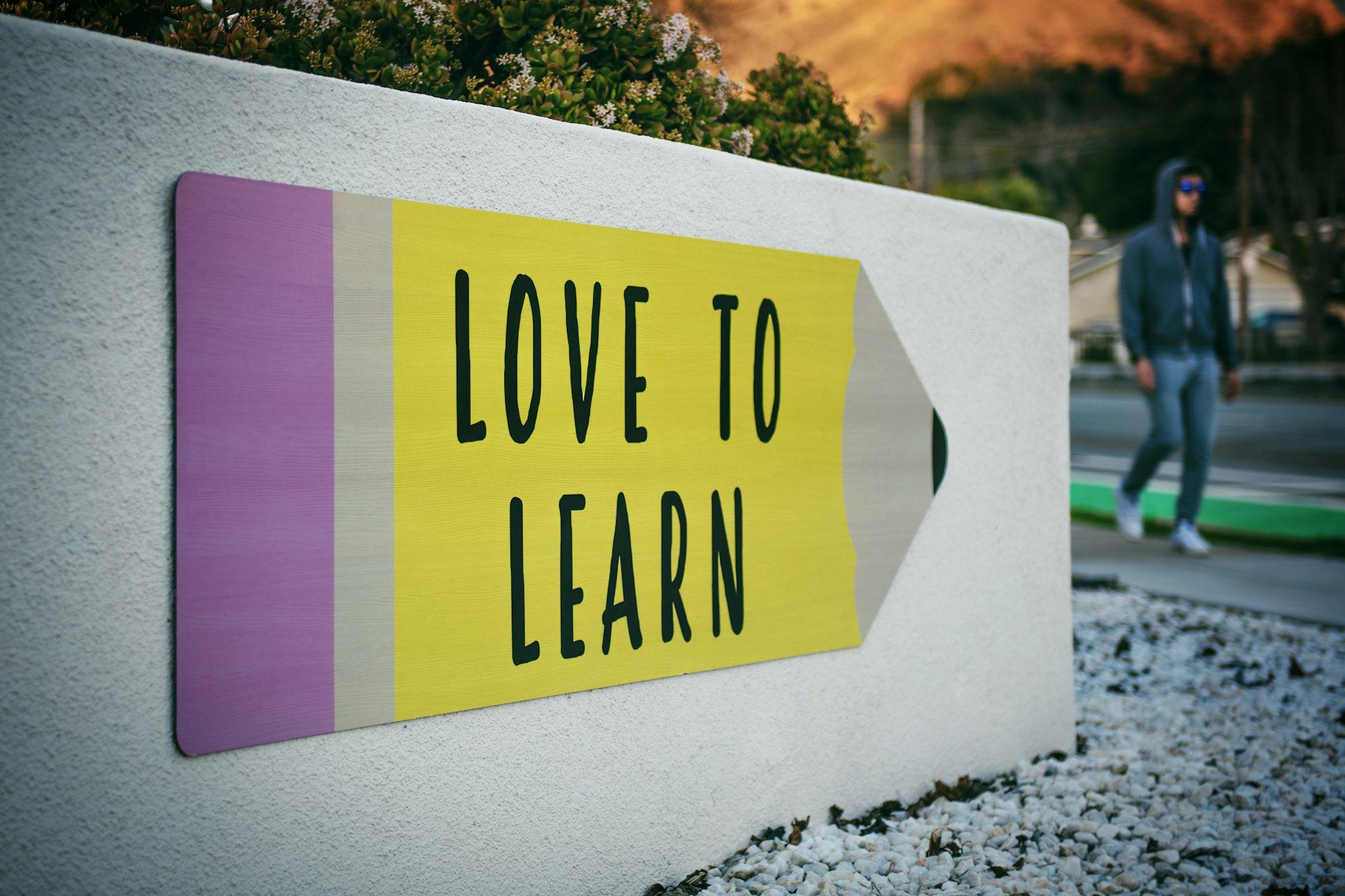
Thanks: Robin
list(map(lambda x: (x in ids), list("123")))
- map()函数
map()是 Python 内置的高阶函数,它接收一个函数 f 和一个 list,并通过把函数 f 依次作用在 list 的每个元素
上,得到一个新的 list 并返回。
map(function, list[])
- list()函数
有一个点要注意:map函数要经过list转换
list(map(int, [1,2,3]))
可以这样写
def fun(p):
return p**2
list(map(fun, [1,2,3]))
传入多个list
list(map(lambda x,y: (x**2, y**3), [1,2,3], [2,3,4]))
[(1, 8), (4, 27), (9, 64)]
any([list1, list2, list3])
- any(iterable)
当any中元素全为0
或者''
或者FALSE
时,返回FALSE,否则返回TRUE
>>> any([0,0,0])
False
>>> any([0,1,2])
True
>>> any((0,))
False
>>> any((0,12))
True
>>> list1 = []
>>> list2 = [1,2,3]
>>> list3 = []
>>> any([list1, list2])
True
>>> any([list1, list3])
False
- all(iterable)
iterable-包含元素的任何可迭代(列表,元组,字典等)
当all中元素全不为0或True时,返回True,否则返回False。
>>> all([0,0,0])
False
>>> all([1,2])
True
>>> all((0,))
False
>>> all((1,))
True
>>> all((0,12))
False
>>> list1 = []
>>> list2 = [1,2,3]
>>> list3 = [2,3]
>>> all([list1, list2])
False
>>> all([list2, list3])
True